Updated: Jul 03, 2023 By: Dessign Team
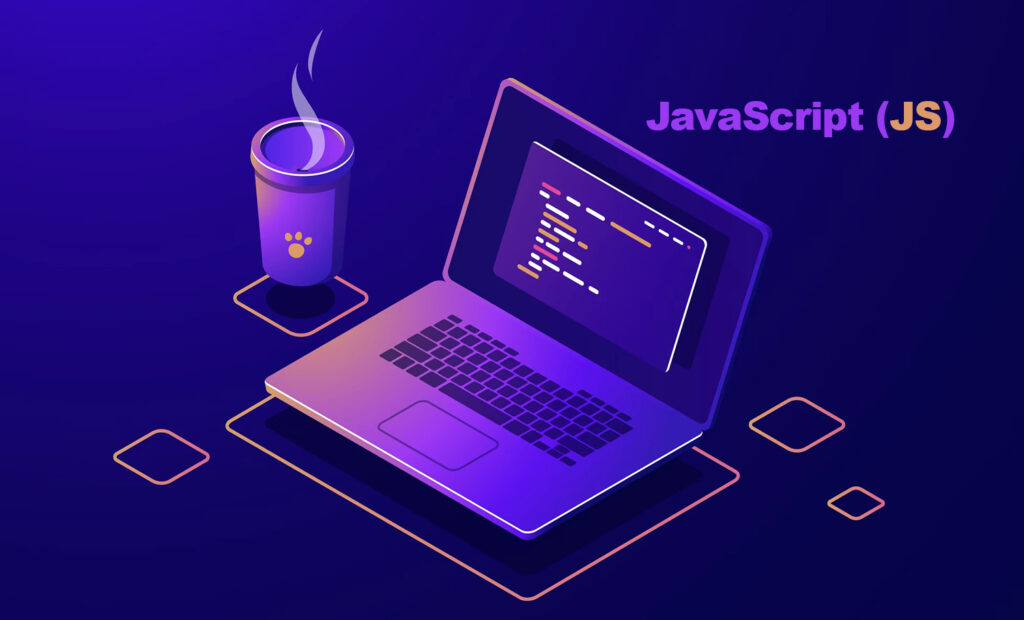
JavaScript is a powerful programming language that is essential for modern web development. Often abbreviated as JS, it is one of the core technologies of the World Wide Web, working alongside HTML and CSS to create dynamic and interactive web pages. With its widespread use, JavaScript has become a crucial element in the development of nearly all web-based applications, as it enables complex features such as realtime content updates, interactive maps, and animated graphics.
The programming language was created by Brendan Eich and has evolved to become highly versatile and beginner-friendly. This adaptability allows JavaScript to cater to various developers’ needs, offering support for object-oriented programming with object prototypes and classes while incorporating elements from other languages like Java and C. Its popularity can be seen in the fact that, as of 2022, 98.7% of websites use JavaScript on the client side for webpage behavior.
Mastering JavaScript opens up opportunities to create immersive online experiences, as it can calculate, manipulate, and validate data while updating and changing both HTML/HTML5 and CSS. This versatility extends to more complex applications such as game development and the creation of animated 2D and 3D graphics, making JavaScript a fundamental building block for web developers across the globe.
JavaScript and HTML/CSS
JavaScript is a scripting or programming language that allows you to implement complex features on web pages, working closely with the other two core technologies of web development, HTML and CSS. HTML provides the structure and content of a webpage, while CSS is responsible for the styling and layout. JavaScript is used to add interactivity and dynamic content to websites, making them more engaging and user-friendly.
DOM Interaction
One essential aspect of JavaScript is its ability to interact with the Document Object Model (DOM). The DOM is a representation of a webpage’s HTML and XML content in a web browser. Through JavaScript, you can manipulate the DOM to add, modify, or delete HTML elements and attributes in real-time, thus allowing web developers to create dynamic, interactive user experiences.
For example, JavaScript can be used to:
- Update the content of an HTML element based on user input
- Add or remove HTML elements in response to user actions
- Change the attributes of HTML elements, such as changing the
src
of an image or thehref
of a link
Styles Modification
In combination with CSS, JavaScript also enables developers to modify the styles of elements on a webpage. By working with CSS classes and style attributes, JavaScript grants developers the power to change element styles directly, offering even more flexibility and control over a webpage’s appearance.
Some key style modifications made possible by JavaScript include:
- Adding, removing or toggling CSS classes to change the look of elements
- Manipulating CSS properties of elements, such as changing colors, sizes, or positioning
- Implementing animations and transitions to create visually engaging effects
Through its interaction with the DOM and modifications of HTML/CSS styles, JavaScript plays a crucial role in the modern web development process. Its capabilities in crafting dynamic, interactive web experiences make it an indispensable tool for any web developer working with HTML and CSS.
Basic Concepts
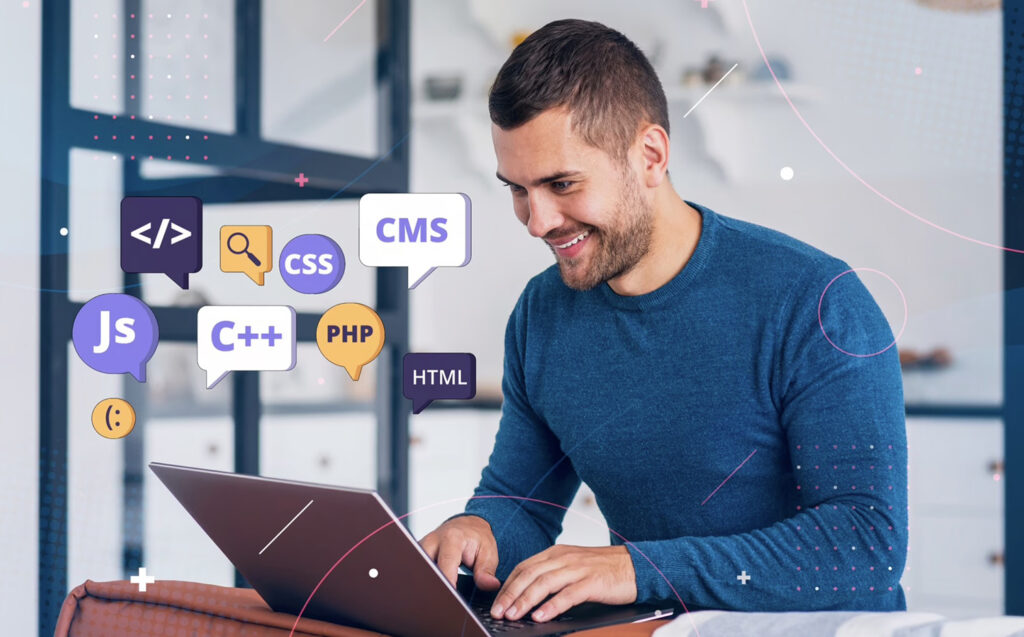
Syntax and Variables
JavaScript is a powerful and versatile programming language, allowing developers to create interactive webpages. The language uses a set of rules, called syntax, for defining instructions to be executed by the computer. To store and manipulate data, JavaScript uses variables, which can be given a name and assigned a value. For example, declaring a simple variable called x
and assigning it a value of 5
is done using the following syntax: var x = 5;
.
Data Types
In JavaScript, there are several basic data types used to represent different types of data. Some of the most common data types include:
- String: Represents a sequence of characters, like text. A string can be created by enclosing a sequence of characters within single or double quotes. For example:
"Hello, World!"
. As in Used in WordPress. - Number: Represents both integers and floating-point numbers. Number examples are
42
or3.14
. - Boolean: Represents either
true
orfalse
. This data type is commonly used in conditional statements and logical operations. - Undefined: A special value assigned to variables that have been declared but not given a value.
- Null: Represents a deliberately empty or non-existent value.
Furthermore, JavaScript also allows developers to create more complex data types, such as functions, objects, and arrays.
- Functions: Reusable blocks of code that can take inputs, perform specific tasks, and return a result.
- Objects: Collections of related data and operations, represented as key-value pairs.
- Arrays: Ordered lists of elements, which can be any JavaScript data type, including other arrays.
Control Structures
Control structures are essential components of a programming language, governing the flow of a program. In JavaScript, there are several common control structures used to dictate the execution and organization of code:
- Conditional statements: Analyze specific conditions using logical operators, such as
if
,else
, andelse if
. These statements determine the flow of a program by executing specific code blocks based on whether a condition is true or false. - Loops: Used for repeated execution of code blocks when a specified condition is satisfied. Common loops in JavaScript include
for
,while
, anddo-while
. - Functions: As mentioned earlier, functions are reusable blocks of code that perform specific tasks. They allow for organizing and modularizing code, making it easier to understand and maintain.
By understanding the syntax, variables, data types, and control structures of JavaScript, you can effectively utilize the capabilities of this versatile programming language to create dynamic and interactive web applications.
Advanced Concepts

Object Oriented Programming
JavaScript is an object-based language, which means it supports key principles of Object Oriented Programming (OOP). These principles include encapsulation, inheritance, and polymorphism. OOP makes it easier to organize and maintain code by grouping related data and functions into objects. In JavaScript, you can use classes to create objects and define their properties and methods.
Built-In Objects
JavaScript comes with various built-in objects that can be leveraged in your programs. Some examples of these objects are String
, Number
, Array
, Date
, and Math
. These objects have properties and methods that can be used to perform operations, manipulate data, and handle complex tasks more efficiently. For example, you can use the Array
object’s push()
method to add an element to an array, or the Date
object’s getFullYear()
method to get the current year.
Creating Custom Objects
In addition to built-in objects, you can also create custom objects to suit your specific programming needs. To create a custom object, you can use object literals, constructors, or classes. Object literals are created using curly brackets and key-value pairs, while constructors and classes involve defining a blueprint for the object and using the new
keyword to create an instance.
Example:
// Object literal
const person =
firstName: "John",
lastName: "Doe",
age: 30,
greet: function()
return `Hello, my name is $this.firstName $this.lastName.`;
;
// Constructor
function Person(firstName, lastName, age)
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
this.greet = function()
return `Hello, my name is $this.firstName $this.lastName.`;
;
const person2 = new Person("Jane", "Doe", 25);
// Class
class Person
constructor(firstName, lastName, age)
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
greet()
return `Hello, my name is $this.firstName $this.lastName.`;
const person3 = new Person("James", "Smith", 40);
Executing Code
JavaScript code can be executed in various ways, such as through browser events, inline scripts, and external script files. Functions are an essential part of executing code in JavaScript, as they allow you to define reusable blocks of code that can be called with specific arguments. One way to define and invoke functions is by using the function
keyword, followed by the function’s name, parameters, and a block of code.
Example:
function add(a, b)
return a + b;
const result = add(5, 3); // 8
Another method for defining functions is using arrow functions, which have a shorter syntax and their own set of rules for handling the this
keyword.
Example:
const multiply = (a, b) => a * b;
const result = multiply(5, 3); // 15
Popular JavaScript Libraries and Frameworks
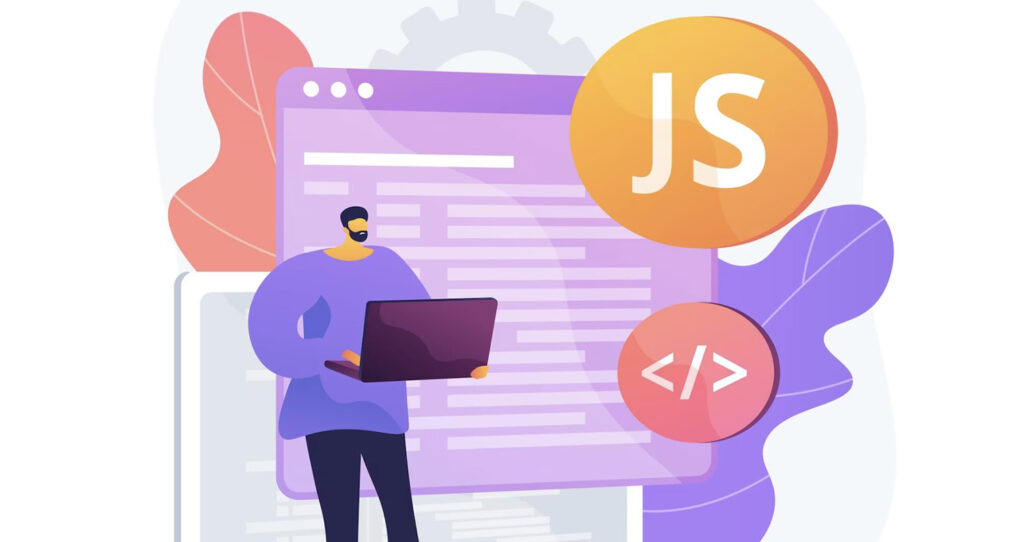
JavaScript has various libraries and frameworks that help developers create interactive and dynamic web applications with ease. In this section, we will discuss three popular JavaScript libraries and frameworks: jQuery, React, and Angular.
jQuery
jQuery is a widely-used JavaScript library that simplifies HTML document traversal, event handling, and animation. It is easy to learn and implement, making it a popular choice among developers who want to manipulate the Document Object Model (DOM) without complex coding. jQuery streamlines tasks such as:
- DOM traversal and manipulation
- Event handling and delegation
- AJAX operations
- Animations and effects
One of the main advantages of using jQuery is its extensive plugin ecosystem, which provides developers with countless solutions for common problems, enhancing productivity and minimizing the amount of custom code they need to write.
React
React is a popular JavaScript front-end library developed by Facebook. It is used to create single-page applications (SPAs) where different components can be rendered efficiently based on incoming data. React’s main features include:
- Component-based architecture: React allows developers to create reusable components, resulting in a more organized and maintainable codebase.
- Virtual DOM: Instead of updating the entire DOM, React creates a “virtual” representation of it, minimizing the number of updates and improving application performance.
- Uni-directional data flow: React supports a top-down data flow, making it easier to manage component states and ensuring that data is consistently passed down to child components.
React has a large community and broad ecosystem, including a variety of third-party libraries and tools that complement its functionality.
Angular
Angular is a powerful JavaScript framework developed by Google for creating scalable and maintainable web applications. It is particularly suited for large and complex projects, thanks to its robust architecture and built-in features. Some key aspects of Angular include:
- Two-way data binding: Angular automatically synchronizes data between the view and the underlying data model, simplifying UI updates and reducing the amount of boilerplate code.
- Dependency injection: Angular’s dependency injection system enables the efficient management of components and their dependencies, promoting modular and easily testable code.
- Directives: With Angular, developers can create custom HTML attributes and elements that add new behaviors to existing DOM elements and improve code readability.
Angular has a steep learning curve due to its comprehensive feature set. However, It is a popular choice among developers for building enterprise-level applications with long-term maintainability and scalability in mind.
JavaScript in Web Browsers
JavaScript plays a significant role in web development, allowing developers to create interactive and dynamic web pages across various browsers such as Chrome, Firefox, Opera, and Edge. This section will explore how JavaScript interacts with web browsers, focusing on interactivity and web pages, browser APIs, and event handling.
Interactivity and Web Pages
JavaScript enables interactivity on web pages by allowing developers to manipulate the Document Object Model (DOM) of a webpage. Through DOM manipulation, elements on a page can be added, removed, or modified, creating engaging user experiences. Furthermore, JavaScript enhances web pages by facilitating various animations, form validation, and dynamic content updates.
JavaScript is supported by all major web browsers, making it a reliable tool to create interactive web pages that work across different platforms.
Browser APIs
Browser Application Programming Interfaces (APIs) empower JavaScript to use various built-in browser functionalities. These APIs include features such as generating 3D graphics, manipulating video streams, accessing geolocation data, and managing cookies. This makes JavaScript a versatile language for both front-end and back-end web development tasks.
Some popular browser APIs are the Geolocation API for obtaining a user’s location, the Web Storage API for storing data in a user’s browser, and the Canvas API for displaying 2D software and 3D graphics.
Event Handling
Event handling is a vital aspect of JavaScript in web browsers, as it enables the detection of user interactions (e.g., clicks, mouse movements, and key presses) and responds to them with appropriate actions. This is facilitated by JavaScript’s ability to associate event listeners with specific HTML elements, causing a function to be executed when the event is triggered.
For example, event handlers can be used to display a pop-up message when a button is clicked, change the color of a text when the mouse hovers over it, or initiate an animation when the user scrolls down the page.
JavaScript’s versatility ensures that web pages remain interactive and engaging, making it an essential component of modern web development.
Server-Side JavaScript: Node.js
Creating a Server
Node.js is an open-source JavaScript runtime environment that allows web developers to run JavaScript code on the server. It is particularly useful for creating web servers, managing data, and interacting with media and storage. To create an HTTP server using Node.js, you can simply utilize its built-in http
module:
const http = require('http');
const server = http.createServer((req, res) =>
res.writeHead(200, 'Content-Type': 'text/plain' );
res.end('Hello, World!');
);
server.listen(3000, () =>
console.log('Server running at http://localhost:3000/');
);
With this basic code, a server is set up to listen on port 3000, and it will respond to all incoming requests with a “Hello, World!” message.
Managing Dependencies
Node.js has a robust package manager called npm
to help developers manage their projects’ dependencies. Npm makes it easy to install and manage third-party packages (libraries or tools) that can enhance your project. For example, to install the popular web framework, Express:
npm install express
This command will download the Express package and add it to the node_modules
folder in your project, thus making it available for use in your code.
Building Web Applications
Building web applications with Node.js often involves utilizing frameworks and middleware to handle common tasks such as routing, templating, and authentication. One widely-used web framework is Express, which simplifies the process of creating server-side web applications.
An example of a basic Express app is as follows:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) =>
res.send('Hello, World!');
);
app.listen(port, () =>
console.log(`Example app listening at http://localhost:$port`);
);
Here, the web application listens on port 3000 and responds with a “Hello, World!” message when the root route (“/”) is accessed.
Using Node.js, web developers can create a wide range of server-side applications, from simple static websites to advanced, data-driven web applications with media and storage capabilities. Its versatility, combined with the familiarity of JavaScript, has made Node.js an indispensable tool in modern web development.
Debugging and Testing
JavaScript developers have many tools at their disposal to ensure that their code is functional, reliable, and bug-free. Debugging tools like Chrome DevTools and Firefox Developer Tools allow developers to inspect and debug code directly in a web browser. They also provide insights into the performance of their code, helping them optimize its speed and efficiency.
Unit testing frameworks, such as Jest and Mocha, help developers write tests that confirm their code is functioning as expected. This is crucial for maintaining high-quality projects.
Optimizing Code Performance
Optimizing JavaScript code is crucial in providing a fast and smooth user experience. Developers can use various techniques to increase the speed of their projects:
- Minification: Tools like UglifyJS and Terser reduce file sizes by removing comments, white space, and other unnecessary elements from the code, thus decreasing the loading time.
- Caching: Caching mechanisms, such as service workers and browser caching, store commonly used resources locally, reducing the need for additional HTTP requests and speeding up load times.
- Using Web Workers: Web Workers allow developers to run JavaScript code in the background, without blocking the main thread, enabling better performance for complex tasks.
Project Structure
A well-structured project is essential for ease of development, code maintainability, and collaboration. Tools such as GitHub provide a platform for developers to collaborate on code, track changes, and manage issues in their projects. When structuring JavaScript projects, developers should:
- Write modular and reusable code, separating the code into different files and functions.
- Use a consistent naming convention for files, variables, and functions.
- Organize project files using a logical folder structure, grouping related files together.
In addition to these best practices, developers can use starter templates, like Create React App and Vue CLI, which provide a pre-configured, best-practice project structure. These tools help developers get started with a new project quickly, focusing on writing their code instead of spending time configuring their environment.
JavaScript Community and Resources
The JavaScript community offers a wealth of resources to help developers learn, hone their skills, and stay up-to-date on the latest trends in the language. In this section, we will explore online tutorials and documentation, popular projects on GitHub, and social media accounts to follow.
Online Tutorials and Documentation
JavaScript has a vast array of tutorials and documentation available to developers at different skill levels. One popular resource is the MDN Web Docs, which provides comprehensive guides and tutorials on the JavaScript language, including its latest version, ECMAScript. Additionally, Codecademy offers interactive JavaScript courses, perfect for beginners and those looking to refresh their skills.
Popular Projects on GitHub
GitHub is home to numerous popular JavaScript projects, allowing developers to explore various libraries and frameworks. These projects can provide inspiration and help to learn by example. Some noteworthy projects include:
- Node.js: A platform built on Chrome’s JavaScript runtime that allows server-side execution of JavaScript code.
- React: A library developed by Facebook for building user interfaces, largely used in web applications.
- AngularJS: A popular framework for building dynamic web applications, backed by Google.
Frequently Asked Questions
How is JavaScript different from other programming languages?
JavaScript is a scripting language primarily used for web development, enabling the creation of dynamic, interactive web content. Unlike many other languages, JavaScript runs directly on browsers, allowing seamless integration with HTML and CSS. Its event-driven, non-blocking nature makes it well-suited for creating responsive, high-performance web applications.
What are the main uses of JavaScript in web development?
In web development, JavaScript is commonly used to add interactivity to websites. It can be used for animations, pop-up windows, search bars, buttons, audio and video playback, chat widgets, and real-time page updates without reloading.
How does JavaScript interact with HTML and CSS?
JavaScript interacts with HTML and CSS by manipulating the Document Object Model (DOM), which represents the structure of a webpage. JavaScript can add, delete, or modify elements within the DOM and change their associated CSS styles, enabling dynamic content and responsive design.
What are some common JavaScript libraries and frameworks?
Popular JavaScript libraries and frameworks include jQuery, an easy-to-use library for DOM manipulation and event handling, React, a component-based library for building user interfaces, Angular, a comprehensive framework for building web applications, and Vue.js, a lightweight, progressive framework for building user interfaces.
How has JavaScript evolved over time?
JavaScript has evolved significantly over its 30-year history. Initially designed as a simple scripting language, major enhancements include the introduction of ECMAScript standards, which dictate new language features and syntax, and the development of Node.js, which allows JavaScript to be used on the server side.
What are primitive data types in JavaScript?
Primitive data types in JavaScript are the most basic data types, including strings, numbers, Booleans, null
, and undefined
. They are immutable, meaning their values cannot be changed, and operations on these values will return new primitive values. More complex data types, like objects and arrays, are not considered primitive because they can be altered and store references rather than the actual values.